Related Blog
In one of my old blogs I tried to explain the similar thing(
Filter OOTB SharePoint ListView web part using IWebPartRow connection Interface.). The purpose of that blog was just show a simple way of using the IWebPartRow connection and a ListView WebPart.
Goal
In the current blog we will try and filter ListView WebPart using Connection object in IWebPartRow. Also there will be a RadioButtonList using which we can drill down the filter.
Create a custom list
- Site Actions > View All Site Content
- Create new List
- Custom List
- Name the list "Sample".
- Visit list settings and add new column "Year".
- Add a few sample data.
- Alter the "All Items" and add ID in the view.
- Now the list will look like below given image.
Web part for Conncetion
- Open Visual Stuido 2010
- Create new project
- Select "Web Part". Don't mistake using "Visual Web Part".
- Rename the WebPart1 to "SampleConnection".
- Use the below given code to be used as a sample.
#region System
using System;
using System.ComponentModel;
using System.Web;
using System.Web.UI;
using System.Web.UI.WebControls;
using System.Web.UI.WebControls.WebParts;
using Microsoft.SharePoint;
using Microsoft.SharePoint.WebControls;
using System.Data;
using System.Collections.Generic;
#endregion
namespace SampleConnection.SampleConnection
{
[ToolboxItemAttribute(false)]
public class SampleConnection : WebPart, IWebPartRow
{
#region Properties
/// <summary>
/// Radiobutton
/// </summary>
private RadioButtonList RbtnYear { get; set; }
/// <summary>
/// Year
/// </summary>
private int XYear
{
get
{
if (System.Web.HttpContext.Current.Request.QueryString["XYear"] == null)
{
return 0;
}
return Convert.ToInt32(System.Web.HttpContext.Current.Request.QueryString["XYear"]);
}
}
/// <summary>
/// The Data table view for the Schema
/// </summary>
private DataTable XTable { get; set; }
#endregion
#region Constructor
/// <summary>
/// Creates the Schema of the table to get filtered column
/// </summary>
public SampleConnection()
{
RbtnYear = new RadioButtonList();
RbtnYear.Items.Insert(0, new ListItem("2004"));
RbtnYear.Items.Insert(1, new ListItem("2007"));
RbtnYear.RepeatDirection = RepeatDirection.Horizontal;
RbtnYear.AutoPostBack = true;
RbtnYear.SelectedIndexChanged += new EventHandler(RbtnYear_SelectedIndexChanged);
//Create the object
XTable = new DataTable();
//Adds the columns which we require for filteration
DataColumn col = new DataColumn();
col.DataType = typeof(int);
col.ColumnName = "Year";
XTable.Columns.Add(col);
//Use the columns to be added to get the Values to be filtered
DataRow row = XTable.NewRow();
if (XYear == 0)
{
row["Year"] = Convert.ToInt32(RbtnYear.Items[0].Value);
}
else
{
row["Year"] = XYear; // Year to be filtered
}
XTable.Rows.Add(row);
}
#endregion
#region Events
/// <summary>
/// Radio Button Events
/// </summary>
/// <param name="sender"></param>
/// <param name="e"></param>
void RbtnYear_SelectedIndexChanged(object sender, EventArgs e)
{
//URL of the Page
string _url = System.Web.HttpContext.Current.Request.Url.AbsolutePath;
_url = string.Format("{0}?XYear={1}", _url, RbtnYear.SelectedValue);
if (System.Web.HttpContext.Current.Request.QueryString.Count > 0)
{
for (int i = 0; i < System.Web.HttpContext.Current.Request.QueryString.Count; i = i + 1)
{
//The xyear
if (string.Compare(System.Web.HttpContext.Current.Request.QueryString.GetKey(i), "XYear", true) == 0)
{
continue;
}
//Recreate the URL
_url = string.Format("{0}&{1}={2}", _url, System.Web.HttpContext.Current.Request.QueryString.GetKey(i), System.Web.HttpContext.Current.Request.QueryString[i]);
}
}
//Send user back to page
System.Web.HttpContext.Current.Response.Redirect(_url);
}
#endregion
#region Methods
/// <summary>
/// Override child controls
/// </summary>
protected override void CreateChildControls()
{
base.CreateChildControls();
//Add the radio button control
this.Controls.Add(RbtnYear);
RbtnYear.SelectedIndex = 0;
if (XYear > 0)
{
if (RbtnYear.Items.FindByValue(XYear.ToString()) != null)
{
RbtnYear.SelectedValue = XYear.ToString();
}
}
}
/// <summary>
/// Connection provider and that assigns the Schema
/// </summary>
/// <returns></returns>
[ConnectionProvider("Row")]
public IWebPartRow GetConnectionInterface()
{
return new SampleConnection();
}
#endregion
#region Implemented property & methods of IWebPartRow
/// <summary>
/// Gets data row view for ListView
/// </summary>
/// <param name="callback"></param>
public void GetRowData(RowCallback callback)
{
callback(XTable.DefaultView[0]);
}
/// <summary>
/// Schema for the ListView web part
/// </summary>
public PropertyDescriptorCollection Schema
{
get
{
return TypeDescriptor.GetProperties(XTable.DefaultView[0]);
}
}
#endregion
}
}
How to use?
- SharePoint Site > Edit a page.
- Click "Add a Web Part".
- Top ribbon > List and Libraries > Select "Sample".
- Again Click "Add a Web Part".
- Top ribbon > Custom > Select "SampleConnection" web part.
- The screen will have now 2 Web Parts. Check out the below given image.
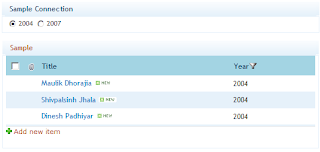
- This happens in Edit Mode of page only, so make sure the page is in Edit Mode. Have a look at the image below to select a connection web part.
- A box will popup to to select a filter. I will select Year. Click "Finish".
- Now to test this stuff. Select the year from the Year from the "Sample Connection" web part.
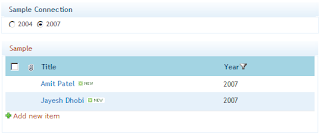
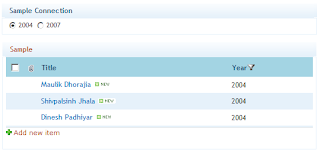
- So this was if a ListView needs filter. If we want to remove it. Just go through step 7 and click "Remove Connection" from the popup box. And the screen will be back to as it was.
Please let me know if this was helpful?
No comments:
Post a Comment